How to Build Smart Contracts Step-by-Step For Blockchain Applications?
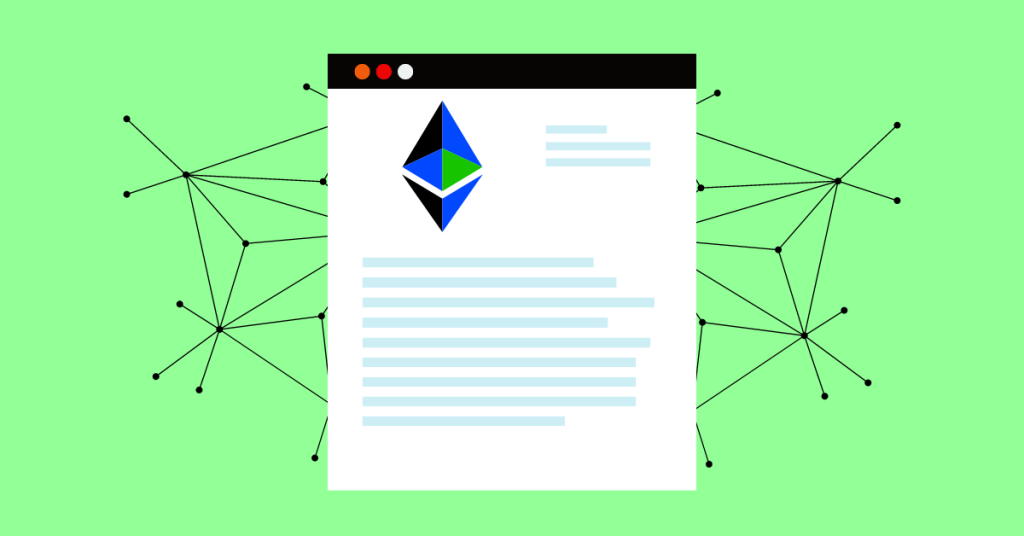
The post How to Build Smart Contracts Step-by-Step For Blockchain Applications? appeared first on Coinpedia Fintech News
Introduction
In the rapidly evolving world of blockchain technology, smart contracts stand out as one of the most transformative innovations. Smart contracts are agreements that run by themselves. The contract’s rules are written as code. These contracts enforce the agreement’s terms when specific conditions are met, without needing middlemen. Smart contracts form the core of blockchain technology.
They allow decentralized applications (dApps) to work without a central authority. Smart contracts ensure transactions and operations are trustworthy, open, and safe by running when conditions are met. Developers who become experts in creating smart contracts can come up with new solutions across many industries.
Smart Contract Development Tools
- Development Environments:
- Truffle: It is a development framework that provides a set of tools for the development, compilation, linking, and deployment of smart contracts.
- Hardhat: Hardhat is a popular choice among developers due to its features like flexibility and extensibility. Also, it has an integrated task runner, and network management ability, and can extend its functionalities through plugins.
- Ganache: A personal blockchain for Ethereum developers. It is used to deploy contracts, develop applications, and run tests.
- Setting up and configuring development environments
1. Install Node.js and setup npm 2. Install Truffle: npm install -g truffle 3. Install Hardhat: install –save-dev hardhat 4. Install Ganache |
Testing Frameworks:
Mocha and Chai are essential for testing the contracts in JavaScript. Mocha is a test framework and Chai is an assertion library both in unison help in running tests and writing test assertions.
Ganache is a personal blockchain for Ethereum development you can use to deploy contracts
Best practices for testing smart contracts:
- Write Comprehensive test cases
- Use Assertions
- Mock External Dependencies
- Automate tests
- Optimize the Gas usage
- Perform security testing
- Maintain a test suite
- Test Network Deployment
Example Usage:
const { expect } = require(“chai”);
describe(“SimpleStorage contract”, function() { await simpleStorage.deployed(); it(“Should store the correct value”, async function() { await simpleStorage.deployed(); const setValue = await simpleStorage.set(42); expect(await simpleStorage.get()).to.equal(42); |
Building Smart Contracts
Smart Contract Lifecycle:
Let’s have a look at the Lifecycle of the Smart contract
1. Design: Here we need to define the design metrics and purpose and requirement of the contract. 2. Development: This is the crucial step where you need to write the code of the contract 3. Testing: We need to validate the contract through testing 4. Deployment: Now once the above steps are done you can deploy the contract to the network. 5. Maintenance: Monitor and update the contract regularly. |
Writing Smart Contracts:
Example of a basic smart contract template (excluding Solidity details):
pragma solidity ^0.8.0;
contract SimpleStorage { function set(uint256 x) public { function get() public view returns (uint256) { |
In the above code, the main components are state variables, functions, and events. Functions in a contract define the actions that need to be performed ad the state variables store the data.
Common Patterns and Practices:
Factory Pattern:
The Factory pattern influences creating many instances of a contract. This comes in handy to create new contracts on the fly, like setting up new versions of a contract for different users or scenarios.
Singleton Pattern:
The Singleton pattern makes sure a contract has just one instance and gives a global way to access it. This proves useful for contracts that handle global state or resources such as a main registry or a contract that governs things.
Best practices for writing maintainable and efficient contracts.
- Keep Contracts Simple and understandable
- Use Descriptive Naming
- Follow Coding standards
- Optimize gas usage
- Implement Access Control
- Conduct Regular Audits
- Maintain proper documentation
Interacting with Smart Contracts:
We use Web3.py, and ether.js for interaction with the deployed contacts and front-end integration
Here are some example code snippets:
from web3 import Web3
# Connect to the Ethereum network # Define the contract ABI and address # Create a contract instance # Call the contract’s functions # Send a transaction to modify the contract’s state |
Following is the code snippet for the frontend integration using Web3,js
<!DOCTYPE html> <html> <head> <title>Simple Storage</title> <script src=”https://cdn.jsdelivr.net/npm/web3@latest/dist/web3.min.js”></script> </head> <body> <h1>Simple Storage</h1> <p id=”storedData”></p> <button onclick=”setData()”>Set Data</button> <script> async function getData() { async function setData() { getData(); |
Smart Contract Security
Security is paramount in Blockchain. Maintaining the security measures and practices is essential.
Common Vulnerabilities:
Some common vulnerabilities in this domain are Reentrancy and Integer Overflow/underflow.
Reentrancy: This is the phenomenon where attackers call a contract repeatedly before the previous execution is completed.
Integer Overflow/Underflow: These are errors occurring when the calculations exceed the maximum or minimum values.
Security Best Practices:
Techniques to secure smart contracts:
- Use the latest Complier version
- Follow the checks-effects-interaction
- Limit the amount of code in fallback functions
- Regularly audit the contracts.
Usage of libraries and frameworks for enhancing security:
OpenZeppelin is a library of secure and community-vetted contracts. It makes sure your contracts are protected.
Auditing Tools:
MythX
MythX is a security analysis tool that performs security scans in your smart contracts.
Steps to integrate MythX into the development workflow:
- Sign up for MythX,
- configure your project
- run scans to identify
- fix security issues.
Slither
Slither looks for bugs in Solidity contracts. It’s a tool that doesn’t run the code but checks it for problems. Slither checks your code to find common safety issues. It also gives ideas to make your code better.
Performing security audits on smart contracts:
- Review the code for vulnerabilities
- Use automated tools
- Address the identifies issues before the final deployment
Deployment and Interaction
Deployment Strategies:
Testnets:
Testnets are blockchain networks that copy the main Ethereum network but use worthless “test” Ether. Developers use them to check smart contracts before they put them on the mainnet.
- Rinkeby: A proof-of-authority testnet that runs and doesn’t break down.
- Ropsten: A proof-of-work testnet that looks a lot like the main Ethereum network.
- Kovan: Another proof-of-authority testnet known to be fast and steady.
Setting up the Testnets for deployment:
//In Truffle module.exports = { networks: { rinkeby: { provider: () => new HDWalletProvider(mnemonic, `https://rinkeby.infura.io/v3/YOUR_INFURA_PROJECT_ID`), network_id: 4, // Rinkeby’s id gas: 4500000, // Rinkeby has a lower block limit than mainnet gasPrice: 10000000000 } ///Deploy the contract: |
At last check the deployed contract on Etherscan or other similar block explorers.
Mainnets
This is the main Ethereum network where all the real life transactions occur and here the deployment involves real ether.
Configuration of the Network:
module.exports = { networks: { mainnet: { provider: () => new HDWalletProvider(mnemonic, `https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID`), network_id: 1, // Mainnet’s id gas: 4500000, // Mainnet’s block limit gasPrice: 10000000000 // 10 gwei (in wei) } } }; |
Gas Optimization Consideration:
Optimizing gas usage is crucial to reduce deployment costs and make your smart contract more efficient.
- Minimize the storage operations
- Conduct Batch operations
- Use efficient data types
- Avoid Dynamic Arrays in storage
Interoperability and Integration
Cross-Chain Compatibility:
Cross-chain interactions are used for interoperability.
Techniques of interacting with multiple blockchain networks:
Atomic Swaps:
Allows the exchange between two different blockchains without involving a third party. Uses the hased-time locked contracts (HTLC) to ensure both parties fulfill the conditions.
Interoperability protocols:
Polkadot and Cosmos allow blockchains to exchange messages freely and interoperate with each other using the Inter-blockchain Communication protocol.
APIs and Oracles:
Oracles serve as third-party services that supply external data to smart contracts. They function as a link between blockchain and off-chain systems enabling smart contracts to engage with real-world data and events.
Why Use Oracles?
Smart contracts can’t access off-chain data or APIs. Oracles provide the external data needed to execute smart contracts based on real-world inputs, which makes them more adaptable and useful.
Chainlink stands out as one of the most used decentralized oracle networks. It allows smart contracts to interact with external data feeds, web APIs, and traditional bank payments.
APIs enable smart contracts to connect with outside services and data boosting what they can do. This includes everything from getting financial info to working with social media sites.
Advanced Topics
Upgradable Smart Contracts:
Proxy Patterns: Proxies allow contract upgrades without changing the contract address.
Techniques to Upgrade: Put into action patterns that let logic updates while keeping data intact.
Layer 2 Solutions:
Layer 2 solutions are used for scalability.
Lightning Network: Bitcoin uses this off-chain fix for quicker cheaper transfers. It sets up payment paths between users.
Plasma and Rollups: Ethereum scales with these tools. They handle trades off-chain and give the main chain a brief recap. This cuts down work for the main blockchain.
Real-World Applications and Case Studies
Case Study Analysis:
We can take the example of Uniswap: Decentralized exchange.
Overview of the project: It is an Ethereum-based DEX using the market maker to facilitate ERC-20 token trading via liquidity pools.
Key Parts: Pools for liquidity, AMM system main contracts contract for creating new pairs contract for routing trades.
Good Practices: regular safety checks, make gas use better, and set up community decision-making with the UNI token.
Project Development:
Guidance on starting and managing your smart contract project:
Define the Project Scope: problem identification and research Choose the right blockchain platform Design the Smart contract: Check the architecture, security and Optimization Develop and Test Deploy and maintain |
Key considerations for project planning and execution.
- User Experience(UX)
- Community engagement
- Regulatory Compliance
- Sustainability.
Conclusion
Before we conclude let’s have a look at the future trends. What’s Coming Up in Smart Contracts?
New tech like Zero-Knowledge Proofs (ZKPs) and Decentralized Autonomous Organizations (DAOs).More use of Layer 2 solutions to scale things up. How This Might Change Future Development and Uses? Smart contracts will keep pushing new ideas in different fields, to make things work better, be more open, and stay safe.
When developers follow these steps and good ways of doing things, they can build, start using, and keep up smart contracts for all blockchain projects. The changing world of blockchain tech opens up exciting opportunities to develop new ideas and build new things. Happy Coding!!
Also Read: Interacting with a Blockchain Network