Interacting with a Blockchain Network
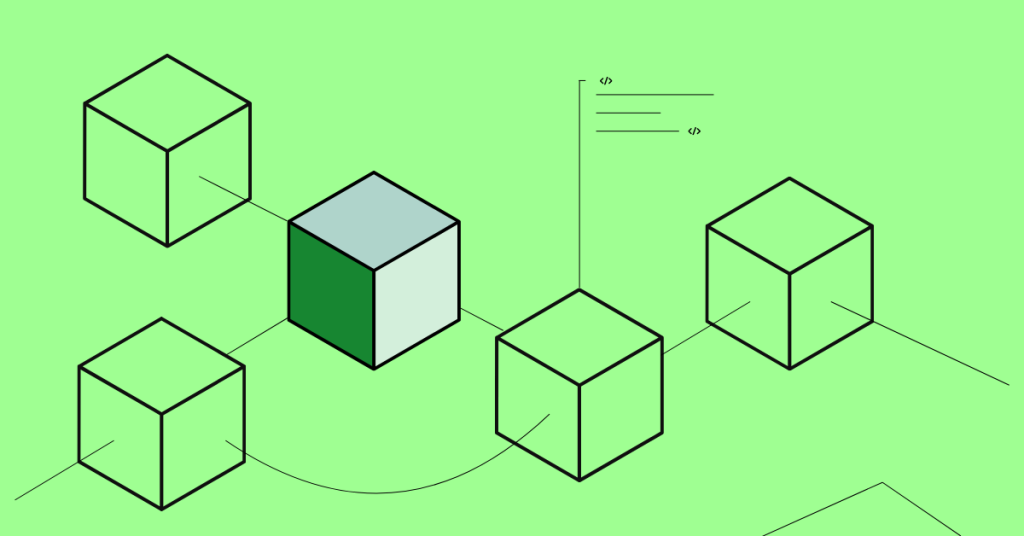
The post Interacting with a Blockchain Network appeared first on Coinpedia Fintech News
1. Introduction
Interaction with blockchain paves the way for developers aiming to leverage blockchain technology. It helps you build decentralized apps, execute smart contracts, and integrate blockchain functionalities. This article provides you, with all the prerequisites and steps needed to set up a suitable environment, perform operations, and develop better solutions and applications in blockchain. So are you ready?
2. Setting Up the Environment
While configuring your environment, it’s essential to choose the right tools according to your interests and requirements.
- Node Connection:
Node connection as the name refers is connecting the node in the network. This node is a gateway to the access of blockchain data and services.
Most of the blockchain nodes provide Remote Procedure Call (RPC) and WebSocket endpoints. Where RPC is mostly used in synchronous requests and Websocket is used in real-time data and event description.
3. Establishing Connections
- Libraries and Tools:
There are various libraries available for establishing connections most of them are based on the two most popular programming languages Python and JavaScript.
JavaScript libraries are Web3.js and ethers.js mostly used for interaction with Ethereum nodes. Web3.py is the equivalent of web3.js in Python which is also used for Ethereum node interactions.
Also, some other libraries are Go-Ethereum based on Golang, and Nethereum based on C#.
Further, for other programming languages, you can check the documentation of various languages and their libraries for configuration.
- API Integration:
Using APIs and libraries to interact with external networks simplifies the interaction. Some popular APIs are Infura which provides scalable infrastructure, Alchemy used for Ethereum development.Infura Infura offers robust infrastructure to link up with the Ethereum network. Infura makes it easy to connect to Ethereum providing dependable and expandable API services. Some other APIs are Quicknode, Moralis, and Cloudflare’s Ethereum gateway.
There are various APIs available but the setup process has the same generic steps as follows:
- Creating an account
- Generating the API key
- Use the generated key to configure your connection.
4. Querying the Blockchain
Querying in blockchain is similar to querying any other database for time-series data. You can request data access to retrieve it and read it.
- Reading Data:
You can get different kinds of information from the blockchain, like block details, transaction data, and account balances. The libraries we talked about before have functions to do read operations. For example, Web3.js has methods such as web3.eth.getBlock() and web3.eth.getTransaction().
- Event Listening:
Blockchain networks create events for specific actions. Setting up listeners lets you respond to these events as they occur in real time. Use WebSocket connections or polling to keep up with the newest events and data and this is a type of handling the data.
5. Writing to the Blockchain
Putting data on a blockchain requires you to create and sign transactions, and work with smart contracts. This section will show you how to do these things using well-known libraries.
- Creating Transactions:
Building and signing transactions:
Javascript(Web3.js)
const Web3 = require(‘web3’);const web3 = new Web3(‘https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID’);
const account = web3.eth.accounts.privateKeyToAccount(‘YOUR_PRIVATE_KEY’); const tx = { web3.eth.sendTransaction(tx) |
Using Web3.py(Python code)
from web3 import Web3 web3 = Web3(Web3.HTTPProvider(‘https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID’)) account = web3.eth.account.privateKeyToAccount(‘YOUR_PRIVATE_KEY’) tx = { ‘from’: account.address, ‘to’: ‘RECIPIENT_ADDRESS’, ‘value’: web3.toWei(0.1, ‘ether’), ‘gas’: 21000, ‘nonce’: web3.eth.getTransactionCount(account.address), signed_tx = account.signTransaction(tx)tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction)receipt = web3.eth.waitForTransactionReceipt(tx_hash)print(receipt) |
Now once you have written the transaction it is sent to the blockchain network for validation and getting included in the block.
JavaScript(Web3.js)
\web3.eth.sendSignedTransaction(signedTx.rawTransaction) |
Python(Web3.py)
tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction)receipt = web3.eth.waitForTransactionReceipt(tx_hash)print(receipt) |
- Smart Contract Interaction:
Dealing with smart contracts that are already up and running means you need to use certain functions to read and change the contract’s saved information(state variables). This back-and-forth lets you tap into everything the smart contract can do making it possible to create complex features in your dApps (decentralized applications).
Interacting with smart contracts:
Configuration: const Web3 = require(‘web3’);const web3 = new Web3(‘https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID’); Reading from the smart contract: Calling a function: Writing: const data = contract.methods.transfer(‘0xRecipientAddress’, web3.utils.toWei(‘1’, ‘ether’)).encodeABI(); const tx = { web3.eth.sendTransaction(tx) |
6. Handling Responses
Handling responses from blockchain interactions the right way is key to creating dependable and easy-to-use apps. This means getting a grip on transaction receipts and figuring out how to parse logs and events that smart contracts generate.
- Transaction Receipts:
Post every transaction a receipt is generated which contains information such as:
- Transaction hash: It is a unique identification code
- Status: Gives the status of transactions as 0 or 1
- Block Number: The block in which the transaction was included
- Gas Used: The amount of gas utilized for the transaction
- Logs: The logs generated by transaction for parsing the event
Example:
tx_hash = web3.eth.sendRawTransaction(signed_tx.rawTransaction) receipt = web3.eth.waitForTransactionReceipt(tx_hash) if receipt[‘status’] == 1: print(‘Transaction successful!’) else: print(‘Transaction failed!’)print(‘Transaction receipt:’, receipt) |
- Logs and Events:
Transactions and smart contracts create logs and events that give useful details about the steps taken and results.
Example: Javascript code
contract.events.MyEvent({ fromBlock: 0 }, (error, event) => { if (error) { console.error(‘Event error:’, error); } else { console.log(‘Event data:’, event); } }); |
7. Security Considerations
Security is the principal of blockchain hence keeping it in consideration is essential.
- Private Keys:
As we know Private keys have restricted access, so safeguarding them is extremely important.You can use hardware wallets or other storage options like AWS KMS, and HashiCorp Vault.
Also never hardcode the value of private keys in your code, always use environment variables or secure vaults.
- Access Control:
Implementing proper access control mechanisms for blockchain interactions is essential. Implement role-based access control and multi-signature wallets to ensure the control and critical interactions are secure.
8. Optimizing Performance
Optimizing the performance in the blockchain is necessary for improving the responsiveness and cost efficiency of the applications.
- Efficient Querying:
Techniques for efficient data querying to reduce latency are
- Batch requests: This means combining multiple requests into one single batch to improve latency.
- Using caching mechanisms: Set up a cache to save often-used information and cut down on repeated queries to the blockchain.
- Gas Optimization:
- Optimize the gas utilized by optimizing the code of your smart contract.
- Use libraries such as OpenZeppelin for optimized functionalities.
- Reduce the cost of the gas used by minimizing the storage used and carrying out batch operations.
9. Testing Interactions
Testing the product is crucial in every development field and so is here, to ensure reliability and functionality.
- Local Test Networks:
Setting up and using local test networks to simulate blockchain interactions:
Ganache for Ethereum setup:
npm install -g ganache-cli ganache-cli const web3 = new Web3(‘http://localhost:8545’); |
- Mocking Blockchain Interactions:
Use Mocking libraries such as Eth-gas-Reporter to track gas usage.
npm install eth-gas-reporter –save-dev
module.exports = { |
10. Continuous Integration and Deployment (CI/CD)
Integrating the blockchain integration tests and automating the deployment enhances the process and improves reliability.
- Automated Testing:
When we talk about automated testing CI/CD pipeline incorporation is inevitable, you can use truffle and hardhat for the same.
- Deployment Automation:
Writing workflows for automated testing and deployment ensures consistent code and helps with quick iterations.
11. Monitoring and Maintenance
- Real-Time Monitoring:
Setting up monitoring tools to track blockchain interactions:
- Prometheus and Grafana: They go hand in hand where Prometheus collects the metrics and Grafana visualizes them.
Following are the steps for the installation:
Install from the official website. Configure:global: scrape_interval: 15s scrape_configs: – job_name: ‘ethereum’ |
- Maintaining Connections:
Ensure persistent and reliable connections to blockchain nodes.Implement a reconnection logic handle the downtime of the node andd maintain the continuous operations aswell.
12. Advanced Topics
- Layer 2 Solutions:
Layer 2 solutions are used for scalability.
Lightning Network: Bitcoin uses this off-chain fix for quicker cheaper transfers. It sets up payment paths between users.
Plasma and Rollups: Ethereum scales with these tools. They handle trades off-chain and give the main chain a brief recap. This cuts down work for the main blockchain.
- Cross-Chain Interactions:
Cross-chain interactions are used for interoperability.
Techniques of interacting with multiple blockchain networks:
- Atomic Swaps:
Allows the exchange between two different blockchains without involving a third party. Uses the hased-time locked contracts (HTLC) to ensure both parties fulfill the conditions.
- Interoperability protocols:
Polkadot and Cosmos allow blockchains to exchange messages freely and interoperate with each other using the Inter-blockchain Communication protocol.
13. Conclusion
The blockchain domain is always changing, with new tools and methods popping up all the time. As you keep going, explore how to customize and improve ways to interact based on what your specific project needs. Keep up with the latest breakthroughs to boost your blockchain development skills and create strong, fault-tolerant decentralized apps. Happy Coding!!
Also Check Out: Understanding Blockchain Networks and Nodes